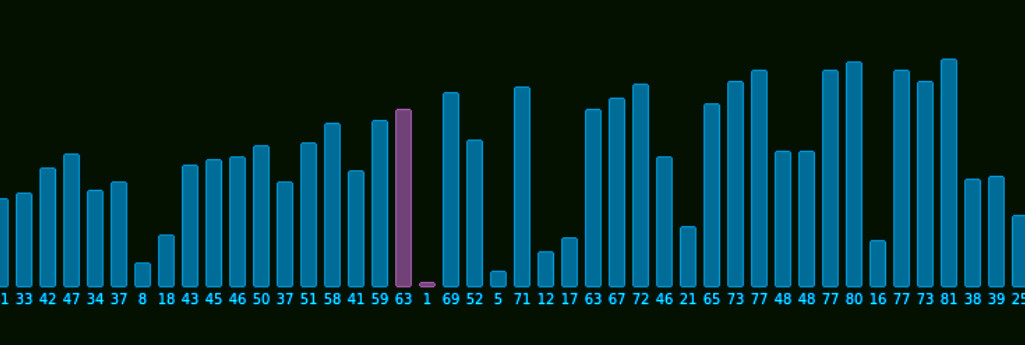

Custom object sorting
Implement a sorting algorithm of your choosing in C++ to arrange your custom objects in an order determined by your code.
Contents
Project specifications
Suggested steps
Keep Coding! Extension exercises
bookProject specification
objective |
Create a custom-typed object that has attributes on which they can be sorted. Design a tool class with a function capable of taking in a pointer to a vector of unsorted objects which then undertakes the sorting process. |
required organs |
Custom classDevise a custom class representing a sortable object of your choosing. The member variables in this class should be private and accessed through public getters and setter methods. Sorting tool classIn a separate class called something like SortingMachine, create a function whose input parameters include a pointer to a vector of your custom type. Test environmentIn what is probably a third class, test your code by creating a vector of your custom type that is unsorted which you then pass into your tool class's SortFoo() function. |
required interface |
Rudimentary menu-based navigation
|
documentation |
Include comments above each function describing its key functionality. Include comments above or a the end of each unclear/important line of code. You should write all the code for your program, copying nothing from any other resource. If you use somebody else's code closely--i.e. using its essential structure but changing variable names--include a citation in your comments. |
sharing |
Get your code working in your repl.it environment and post a link to the file including your main() method in the project tracker tab of our master work spreadsheet. |
Suggested Steps
STEP 1: Create a class representing your chosen collectible object that includes private members and public accessor methods and a constructor
STEP 2: Use your class in a simple program and create a vector of several of your objects for use in our sorting exercise next week.
STEP 3: Demonstrate as we did in class on Wednesay, that you in fact have multiples different objects with different member variables.
STEP 4: Begin the design process by tinkering with the bubble sort visualization linked at left, and relying only on pseudocode, not other folks' C++
IDEA: Investigate (try in documentation, not stack overflow) how to randomly create values for the various objects in your vector.
STEP 5: Create your tool class and its internal method for sorting a vector of your custom object.
Start with a simple sort
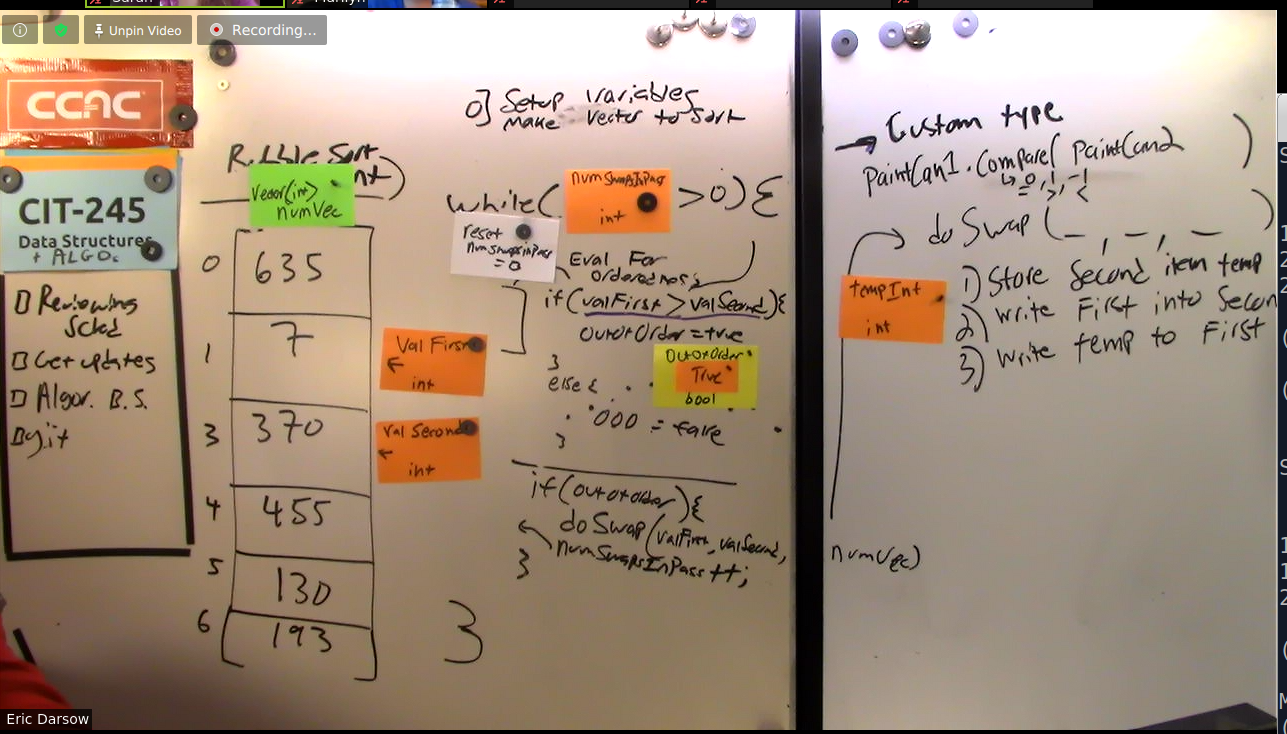
Extension ideas
Implement additional sort algorithms
Bubble sort is one of the classic sorting algos, so start with that, but others exist that are much more efficient, such as Quick sort or merge sort. Implement one of these.
Compare sort algorithm efficiency
If you feel ambitious, access the system clock and time how long the sorting takes between your two algorithms. This will likely involve creating a hella big list of objects so the time differences are measurable at the millisecond level.