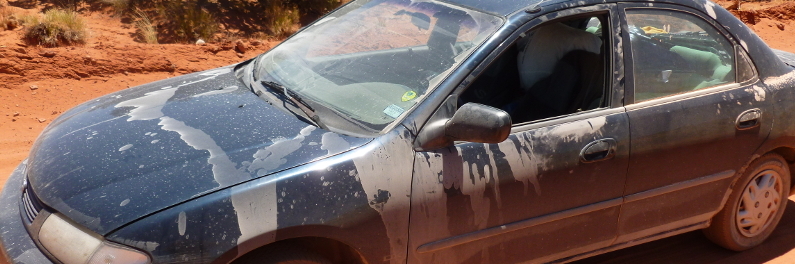
Python Road Trip: Variables and conditional logic in python
Explore python fundamentals with exercises that involve travel calculations such as gas mileage, travel costs, and more.
Learning Objectives
check_box | Create variables in python and initialize them with both hard-coded values and user-input. |
---|---|
check_box | Use essential mathematical opeators to manipulate variables of varying types. |
Part 1: Hard-coding variable and using basic operators
- Create a new python file called roadtrip.py using either in your IDE or using repl.it
- Create a comment block at the top of your file (using # characters) that contains your name, the course name, and the purpose of this code: to demonstrate variable assignment operations and basic operators
- Write a python expression that displays the text "Road trip efficiency comparisons"
- Declare a variable named small_vehicle and assign to that variable a string describing the year, make, and model of a small car you find interesting. This can be a hybrid or an EV or a regular old ICE car. For example you could assign the string "1996 Mazda Protege" to small_vehicle
- Choose a large_vehicle year, make, and model and declare that variable and assign to it a string describing your vehicle of choice.
- Navigate to the EPA's official mileage efficiency listings for vehicles on American roads. Look up the year, make, and model of your small car and large car variables.
- Declare two variables called small_vehicle_combined_mpg and large_vehicle_combined_mpg and assign to them the EPA's combined mileage rate
- Create two more variables of appropriate names that contain the URL to a website featuring a photo of your chosen vehicles. For example, if you chose the Ford Maverick pickup, you could have a variable called large_car_url and assign to it "https://www.ford.com/trucks/maverick/"
- Now write a set of print() expressions that display to the console the basic facts about each of your chosen vehicles. The output should look something like the clip below. Remember, don't retype the vehicle information or mileage rates. Rather, access the variables you made in your print() statements.

Part 2: Compute road trip metrics
- Divide the output from part 1 from this output by printing out a row of some dashes like ----------------
- Prompt the user of your program with a printed statement to enter the total miles of a proposed road trip.
- Then read into a variable of a sensible name the number of miles of the trip. Your variable could be called trip_miles. Immediately cast the user's input to a float (a number with a decimal point) with code like this trip_miles = float(input())
- Compute the total number of gallons of gasoline required to undertake a trip of the inputted number of miles in both your small and large vehicles. Do this by writing two python expressions, each of which uses the = assignment operator to store the result of dividing trip length by miles per gallon in the corresponding variable on the left. Your new variables can be called something like small_vehicle_gallson and large_vehicle_miles. For example: small_vehicle_gallons = trip_miles / small_vehicle_combined_mpg
- Display the results of your two gallon requirements in two additional lines of output, one for each car, that outputs the vehicle type, the trip length, and the gallons of gas required.
- Compute how many more gallons the large car will require than the small car. Use the subtraction operator and store the result of your subtraction in a sensibly named variable. Display the result after your gallon requirements are displayed.
- Use the sample output below to help guide your coding.

Part 3: Fuel costs and expected travel times
- Print another line of dashes to separate out part 2 from this part 3
- Ask the user for the average cost of a gallon of gasoline expected on the trip.
- Now use the float(input()) pattern as in part 2 to read in the price per gallon of gasoline into a sensibly named variable.
- Compute the total trip cost for each vehicle using the multiplication operator and the basic expression that total cost of trip = gallons of fuel required x price per gallon of fuel. Use sensibly named variables to store your result.
- Just like in part 2, create three lines of output: one line displaying the total expected trip cost for each vehicle and the third line comparing the difference in trip costs.
- Create two hard-coded variables that store the expected driving speed of cars on two types of roads: US interstate highways (the big, access-controlled roads) and local roads.
- Optional extension: Ask the user for their expected speeding adjustment rate for each type of road. For example, some folks basically always drive 11 miles per hour over the posted speed limit on interstates and 5 over on local roads. Read these values into their own variables. Then compute two "actual speed" variables that add the hard-coded speeds per road type to the user's speeding buffers.
- Compute expected travel time for the trip of the given length on both types of road, taking into account the expected speeding. Travel time can be calculated by dividing total trip miles by travel rates in miles per hour.
- Display the results of your travel time computations for each type of car on each type of road.
Link to code
For reference, here's some help code for the above exercises.
Extension exercises
For students who have python experience or new programmers with lots of gusto, implement one or more of these features in our road trip program.
Add conditional logic for warnings
- Implement conditional logic to display an alert if either of the program's cars achieve less than 20 miles per gallon of efficiency. If both cars get over 20 miles per gallon, display a thank you phrase on behalf of the global climate.
- Implement conditional logic that displays "multi day trip" if the user enters a trip distance that reasonably would require a driver longer than a day of driving to complete. If the trip would require less than a day, display that instead. If the trip would likely require less than an hour of driving, print that out to the user.
Implement with dictionaries
- Create a dictionary that is keyed by the string name of the year, make, and model of a car. Write the value of each key as the combined mileage for that vehicle. Maintain your hard-coded variables called small_vehicle, etc. and use them to populate your new dictionary. Write a for loop that displays this data in a pretty printed fashion.
- Write a simple user interface that allows the user the program to enter a year, make, and model and then enter the combined MPG for the car the just entered. Write those two user inputs as key-value pairs into your dictionary. Then display all the K-V pairs.
- Ask the user for a road trip distance. Store that value. Then loop over all the cars in your dictionary and display the basic info about that vehicle followed by the number of gallons of gas required to undertake the trip of the given distance. Try to pretty print the data using tab characters so the mileage rates and gas requirements print in column format.
Carbon footprints of various modes of travel
Find a tool that compares the carbon output of various modes of travel such as this one by sustainable travel international. The modify your program to not only display how many gallons of gas are required to undertake a trip but also displays the total weight of carbon that trip with that car will input into the atmosphere. You could also try asking the user for a total trip distance and then provide a comparison of the carbon output to undertake that trip in a vehicle versus an airliner versus a boat of some kind.